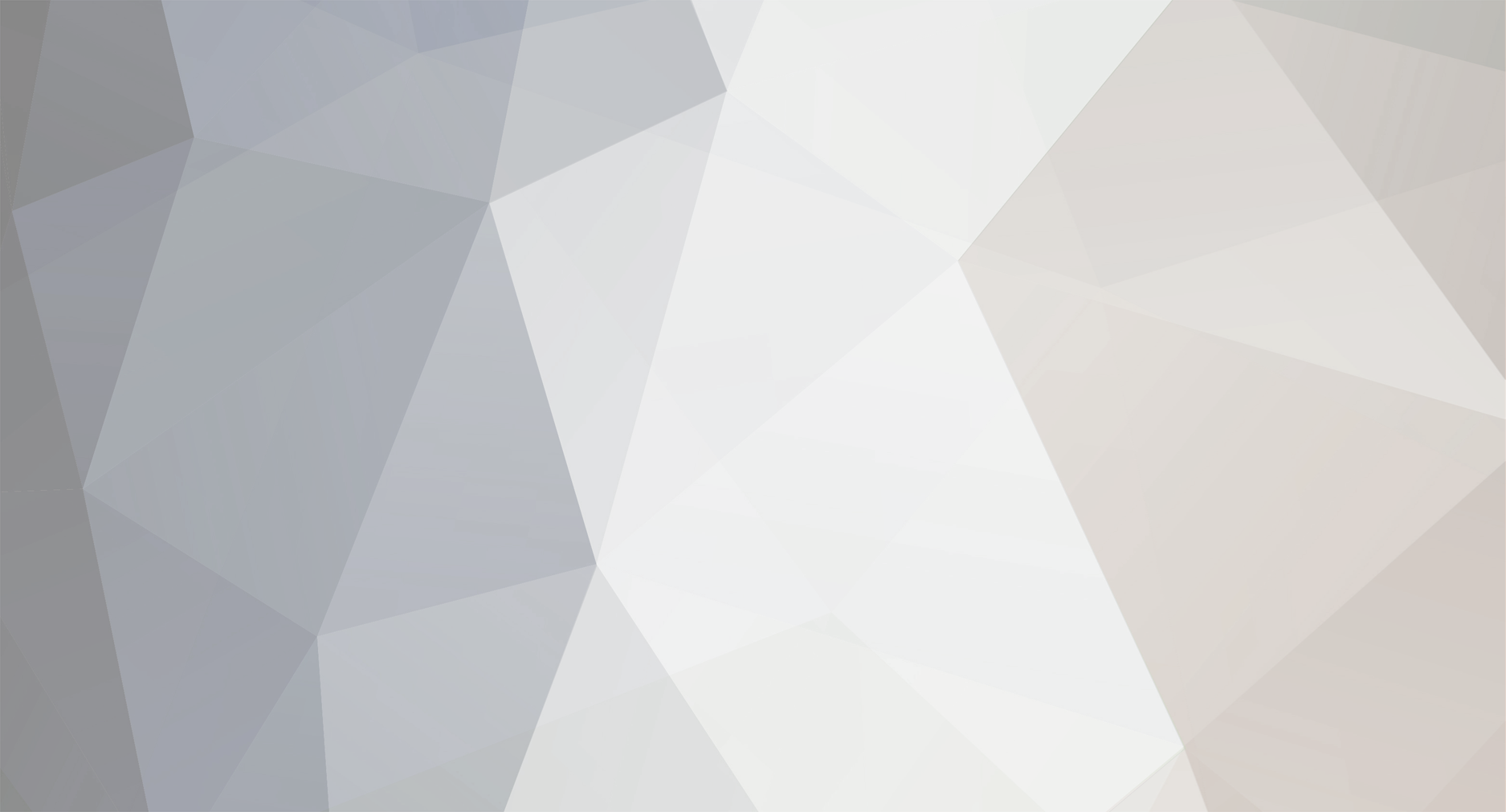
Prosper
Members-
Posts
331 -
Joined
-
Last visited
-
Days Won
1
Content Type
Profiles
Forums
Blogs
Everything posted by Prosper
-
This removes and replaces std::string with my own. http://codepad.org/AWPaMm6U http://codepad.org/xuRpWhkr (slight update, I make sure a null terminator is set after input is grabbed)
-
For multiplication. Multiplication of course can be even slower if you pick high enough values. http://codepad.org/fd1ijnze #include <iostream> #include <string> using namespace std; unsigned int SymbolToNativeIndex(unsigned char chVal) { unsigned int nVal = 0; if (chVal == '0') { nVal = 0; } else if (chVal == '1') { nVal = 1; } else if (chVal == '2') { nVal = 2; } else if (chVal == '3') { nVal = 3; } else if (chVal == '4') { nVal = 4; } else if (chVal == '5') { nVal = 5; } else if (chVal == '6') { nVal = 6; } else if (chVal == '7') { nVal = 7; } else if (chVal == '8') { nVal = 8; } else if (chVal == '9') { nVal = 9; } return nVal; } unsigned char GetNext(unsigned char chVal) { unsigned char chValB = chVal; if (chValB == '0') { chValB = '1'; } else if (chValB == '1') { chValB = '2'; } else if (chValB == '2') { chValB = '3'; } else if (chValB == '3') { chValB = '4'; } else if (chValB == '4') { chValB = '5'; } else if (chValB == '5') { chValB = '6'; } else if (chValB == '6') { chValB = '7'; } else if (chValB == '7') { chValB = '8'; } else if (chValB == '8') { chValB = '9'; } else if (chValB == '9') { chValB = '0'; } return chValB; } unsigned char GetNextDown(unsigned char chVal) { unsigned char chValB = chVal; if (chValB == '0') { chValB = '9'; } else if (chValB == '1') { chValB = '0'; } else if (chValB == '2') { chValB = '1'; } else if (chValB == '3') { chValB = '2'; } else if (chValB == '4') { chValB = '3'; } else if (chValB == '5') { chValB = '4'; } else if (chValB == '6') { chValB = '5'; } else if (chValB == '7') { chValB = '6'; } else if (chValB == '8') { chValB = '7'; } else if (chValB == '9') { chValB = '8'; } return chValB; } std::string InitBlankNumber() { std::string strVal; strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); return strVal; } // numbers must be of fixed size 10 characters // 0000000000 to 9999999999 (expected input range) std::string GetNextNumber(std::string strNumber) { std::string strVal; strVal = InitBlankNumber(); unsigned char chIndex = '9'; if (strNumber[SymbolToNativeIndex(chIndex)] != '9') { strVal[SymbolToNativeIndex(chIndex)] = GetNext(strNumber[SymbolToNativeIndex(chIndex)]); chIndex = GetNextDown(chIndex); while (chIndex != '0') { strVal[SymbolToNativeIndex(chIndex)] = strNumber[SymbolToNativeIndex(chIndex)]; chIndex = GetNextDown(chIndex); } strVal[SymbolToNativeIndex(chIndex)] = strNumber[SymbolToNativeIndex(chIndex)]; } else if (strNumber[SymbolToNativeIndex(chIndex)] == '9') { strVal[SymbolToNativeIndex(chIndex)] = '0'; chIndex = GetNextDown(chIndex); bool bStillNine = true; while (bStillNine == true) { bStillNine = (strNumber[SymbolToNativeIndex(chIndex)] == '9'); if (bStillNine == true) { strVal[SymbolToNativeIndex(chIndex)] = '0'; } else { strVal[SymbolToNativeIndex(chIndex)] = GetNext(strNumber[SymbolToNativeIndex(chIndex)]); chIndex = GetNextDown(chIndex); bStillNine = false; break; } chIndex = GetNextDown(chIndex); if (chIndex == '0' && bStillNine == true) { if (strNumber[SymbolToNativeIndex(chIndex)] == '9') { strVal[SymbolToNativeIndex(chIndex)] = '0'; } else { bStillNine = false; } break; } } while (chIndex != '0') { strVal[SymbolToNativeIndex(chIndex)] = strNumber[SymbolToNativeIndex(chIndex)]; chIndex = GetNextDown(chIndex); } if (chIndex == '0' && bStillNine == false) { strVal[SymbolToNativeIndex(chIndex)] = strNumber[SymbolToNativeIndex(chIndex)]; } } return strVal; } std::string AddNumberToNumber(std::string l, std::string r) { std::string strResult = l; std::string strCounter = InitBlankNumber(); while (strCounter != r) { strResult = GetNextNumber(strResult); strCounter = GetNextNumber(strCounter); } return strResult; } std::string MultiplyNumberByNumber(std::string l, std::string r) { std::string strResult = InitBlankNumber(); std::string strCounter = InitBlankNumber(); while (strCounter != r) { strResult = AddNumberToNumber(strResult, l); strCounter = GetNextNumber(strCounter); } return strResult; } std::string GetInput(); void Test(); int main() { Test(); return 0; } std::string GetInput() { std::string strInput; getline(cin, strInput); return strInput; } void Test() { while (true) { cout << "Input value A: " << endl; std::string strA = GetInput(); if (strA.size() != 10) { strA = InitBlankNumber(); cout << "Number should only be length of 10. Value will be default of: " << InitBlankNumber() << endl; } cout << "Input value B: " << endl; std::string strB = GetInput(); if (strB.size() != 10) { strB = InitBlankNumber(); cout << "Number should only be length of 10. Value will be default of: " << InitBlankNumber() << endl; } //cout << AddNumberToNumber(strA, strB) << endl; cout << MultiplyNumberByNumber(strA, strB) << endl; cout << "Press q to quit otherwise input something else to continue." << endl; if (GetInput() == "q") { break; } } cout << "Any key plus enter to exit." << endl; GetInput(); }
-
I wanted to write a program that can add numbers. I avoided using built in addition and for loops . I avoided less than and greater than signs. I did use however equal to signs and not equal to signs. If the forum moderators can guarantee this topic won't be lost I will permit them to move it to developer's corner. I notice developer's corner topics fade away. WARNING: Try to avoid the first 2 to 3 digits in the second number unless you're willing to wait for hours or maybe a day. http://codepad.org/FZ1zXFNb #include <iostream> #include <string> using namespace std; unsigned int SymbolToNativeIndex(unsigned char chVal) { unsigned int nVal = 0; if (chVal == '0') { nVal = 0; } else if (chVal == '1') { nVal = 1; } else if (chVal == '2') { nVal = 2; } else if (chVal == '3') { nVal = 3; } else if (chVal == '4') { nVal = 4; } else if (chVal == '5') { nVal = 5; } else if (chVal == '6') { nVal = 6; } else if (chVal == '7') { nVal = 7; } else if (chVal == '8') { nVal = 8; } else if (chVal == '9') { nVal = 9; } return nVal; } unsigned char GetNext(unsigned char chVal) { unsigned char chValB = chVal; if (chValB == '0') { chValB = '1'; } else if (chValB == '1') { chValB = '2'; } else if (chValB == '2') { chValB = '3'; } else if (chValB == '3') { chValB = '4'; } else if (chValB == '4') { chValB = '5'; } else if (chValB == '5') { chValB = '6'; } else if (chValB == '6') { chValB = '7'; } else if (chValB == '7') { chValB = '8'; } else if (chValB == '8') { chValB = '9'; } else if (chValB == '9') { chValB = '0'; } return chValB; } unsigned char GetNextDown(unsigned char chVal) { unsigned char chValB = chVal; if (chValB == '0') { chValB = '9'; } else if (chValB == '1') { chValB = '0'; } else if (chValB == '2') { chValB = '1'; } else if (chValB == '3') { chValB = '2'; } else if (chValB == '4') { chValB = '3'; } else if (chValB == '5') { chValB = '4'; } else if (chValB == '6') { chValB = '5'; } else if (chValB == '7') { chValB = '6'; } else if (chValB == '8') { chValB = '7'; } else if (chValB == '9') { chValB = '8'; } return chValB; } std::string InitBlankNumber() { std::string strVal; strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); strVal.push_back('0'); return strVal; } // numbers must be of fixed size 10 characters // 0000000000 to 9999999999 (expected input range) std::string GetNextNumber(std::string strNumber) { std::string strVal; strVal = InitBlankNumber(); unsigned char chIndex = '9'; if (strNumber[SymbolToNativeIndex(chIndex)] != '9') { strVal[SymbolToNativeIndex(chIndex)] = GetNext(strNumber[SymbolToNativeIndex(chIndex)]); chIndex = GetNextDown(chIndex); while (chIndex != '0') { strVal[SymbolToNativeIndex(chIndex)] = strNumber[SymbolToNativeIndex(chIndex)]; chIndex = GetNextDown(chIndex); } strVal[SymbolToNativeIndex(chIndex)] = strNumber[SymbolToNativeIndex(chIndex)]; } else if (strNumber[SymbolToNativeIndex(chIndex)] == '9') { strVal[SymbolToNativeIndex(chIndex)] = '0'; chIndex = GetNextDown(chIndex); bool bStillNine = true; while (bStillNine == true) { bStillNine = (strNumber[SymbolToNativeIndex(chIndex)] == '9'); if (bStillNine == true) { strVal[SymbolToNativeIndex(chIndex)] = '0'; } else { strVal[SymbolToNativeIndex(chIndex)] = GetNext(strNumber[SymbolToNativeIndex(chIndex)]); chIndex = GetNextDown(chIndex); bStillNine = false; break; } chIndex = GetNextDown(chIndex); if (chIndex == '0' && bStillNine == true) { if (strNumber[SymbolToNativeIndex(chIndex)] == '9') { strVal[SymbolToNativeIndex(chIndex)] = '0'; } else { bStillNine = false; } break; } } while (chIndex != '0') { strVal[SymbolToNativeIndex(chIndex)] = strNumber[SymbolToNativeIndex(chIndex)]; chIndex = GetNextDown(chIndex); } if (chIndex == '0' && bStillNine == false) { strVal[SymbolToNativeIndex(chIndex)] = strNumber[SymbolToNativeIndex(chIndex)]; } } return strVal; } std::string AddNumberToNumber(std::string l, std::string r) { std::string strResult = l; std::string strCounter = InitBlankNumber(); while (strCounter != r) { strResult = GetNextNumber(strResult); strCounter = GetNextNumber(strCounter); } return strResult; } std::string GetInput(); void Test(); int main() { Test(); return 0; } std::string GetInput() { std::string strInput; getline(cin, strInput); return strInput; } void Test() { while (true) { cout << "Input value A: " << endl; std::string strA = GetInput(); if (strA.size() != 10) { strA = InitBlankNumber(); cout << "Number should only be length of 10. Value will be default of: " << InitBlankNumber() << endl; } cout << "Input value B: " << endl; std::string strB = GetInput(); if (strB.size() != 10) { strB = InitBlankNumber(); cout << "Number should only be length of 10. Value will be default of: " << InitBlankNumber() << endl; } cout << AddNumberToNumber(strA, strB) << endl; cout << "Press q to quit otherwise input something else to continue." << endl; if (GetInput() == "q") { break; } } cout << "Any key plus enter to exit." << endl; GetInput(); }
-
I'm abandoning this project. The source code is annoying. And i have more direct ways to do what I want.
-
Good catch men! Also I was modding to 9 which gives <= 8. But 8 is an index that accesses outside of an 8 length array. I made some other mistakes too. Here's the source code also runs with irrlicht3d now. http://codepad.org/F7qOhi8j Also changed acceleration to have a variable offset for each axis. amendAcceleration no longer adds onto existing acceleration value. Many other things have changed too. A video showing the particles.
-
http://codepad.org/8Gt6vuVB Figured it out. Took me a while.
-
I'm not exactly sure what you're asking for feedback on here Also, your simulation is taking huge time steps. You're sampling 5 times per reset, which, if your purpose is to gather data, is not very many samples. Thanks Burke! Didn't account for unit size where I should have. There are many other problems too. Like modulolololing to 8 rather than 9. but further more if i have 6 faces each with 8x8 grid the 6x8x8 booleans are enough but i have no idea how to properly place a point like that. it be better if i went 8x8x8 first. With this addition I am no longer just checking the faces of the cube for a collision. I will look into transforming into 6x8x8. http://codepad.org/k2KFEhhV
-
I create particles with random positions and accelerations. I set mass too but won't be using it for a while. So after giving the particle a position and an acceleration it accelerates from its position. At every frame after i've collected data from the faces of the cube, I have to clear the faces of the cube by reseting their values to false. Every face has an 8x8 grid of boolean values that are triggered true depending on if a particle hits . Only the parts of the grid that are hit are triggered true. I give 5 seconds for the particles do what they are going to do, then i remove them and make a new particle list. The reason i haven't enabled collision now is I like to watch them pass through each other, if they collided you get totally different results. Collisions can be costly too. BUT MEN YOU SAY, WHAT IS THE PURPOSE? By extracting particle information from space-time in the way I have, I hope to use the bit states to feed another application. Such as shoveling the bits as bytecode through a VM causing potential functionality. If I get really good i might be able to do to things at once. Not just a physics simulation but also get other things accomplished. I'm taking in PRNG data right now, but you can imagine how adjusting the seeds at the right 5 second intervals might just be enough for me to succeed. Here is the source code. #include <iostream> #include <vector> using namespace std; unsigned int xor128() { static unsigned int x = 8584732; static unsigned int y = 6525464; static unsigned int z = 3711115; static unsigned int w = 9032831; unsigned int t; t = x ^ (x << 11); x = y; y = z; z = w; return w = w ^ (w >> 19) ^ (t ^ (t >> ); } unsigned int GetRandomNumber() { return xor128(); } bool*** g_Cells = 0; const unsigned int g_nSquaresInARow = 8; const unsigned int g_nCenterOfARow = 4; const unsigned int g_nFaces = 6; const unsigned int g_nUnitSize = 10; void InitCells() { g_Cells = new bool**[g_nFaces]; for (unsigned int yyy = 0; yyy < g_nSquaresInARow; ++yyy) { g_Cells[yyy] = new bool*[g_nSquaresInARow]; for (unsigned int xxx = 0; xxx < g_nSquaresInARow; ++xxx) { g_Cells[yyy][xxx] = new bool[g_nSquaresInARow]; for (unsigned int iii = 0; iii < g_nSquaresInARow; ++iii) { g_Cells[yyy][xxx][iii] = false; } } } } void DeInitCells() { for (unsigned int yyy = 0; yyy < g_nSquaresInARow; ++yyy) { for (unsigned int xxx = 0; xxx < g_nSquaresInARow; ++xxx) { delete [] g_Cells[yyy][xxx]; g_Cells[yyy][xxx] = 0; } } for (unsigned int yyy = 0; yyy < g_nSquaresInARow; ++yyy) { delete [] g_Cells[yyy]; g_Cells[yyy] = 0; } delete [] g_Cells; g_Cells = 0; } void ResetCells() { for (unsigned int zzz = 0; zzz < g_nFaces; ++zzz) { for (unsigned int yyy = 0; yyy < g_nSquaresInARow; ++yyy) { for (unsigned int xxx = 0; xxx < g_nSquaresInARow; ++xxx) { g_Cells[zzz][yyy][xxx] = false; } } } } struct Point3D { unsigned int X; unsigned int Y; unsigned int Z; Point3D(); }; Point3D::Point3D() : X(g_nCenterOfARow), Y(g_nCenterOfARow), Z(g_nCenterOfARow) { } class Object { public: Object(); void setPos(Point3D position); Point3D getPos(); void update(); void amendAcceleration(signed int nVal); void setMass(unsigned int nVal); unsigned int getMass(); private: Point3D m_position; unsigned int m_nMass; signed int m_nAcceleration; }; Object::Object() : m_nMass(1), m_nAcceleration(1) { } void Object::setPos(Point3D position) { m_position = position; } Point3D Object::getPos() { return m_position; } void Object::update() { m_position.X = m_position.X + m_nAcceleration; m_position.Y = m_position.Y + m_nAcceleration; m_position.Z = m_position.Z + m_nAcceleration; } void Object::amendAcceleration(signed int nVal) { m_nAcceleration = m_nAcceleration + nVal; } void Object::setMass(unsigned int nVal) { m_nMass = nVal; } unsigned int Object::getMass() { return m_nMass; } std::vector<Object> g_objects; void MakeObjects() { for (unsigned int iii = 0; iii < 25; ++iii) { Object object; Point3D point; point.X = GetRandomNumber(); point.Y = GetRandomNumber(); point.Z = GetRandomNumber(); object.setPos(point); object.setMass(GetRandomNumber() % ; signed int nVal = GetRandomNumber() % 3; if (GetRandomNumber() % 2 == 1) { nVal = -nVal; } object.amendAcceleration(nVal); } } void BoundaryCheck(Point3D point) { unsigned int maxZ = g_nUnitSize * g_nSquaresInARow; unsigned int minZ = 0; unsigned int maxY = g_nUnitSize * g_nSquaresInARow; unsigned int minY = 0; unsigned int maxX = g_nUnitSize * g_nSquaresInARow; unsigned int minX = 0; if (point.Z >= maxZ || point.Z <= minZ) { } else if (point.Y >= maxY || point.Y <= minY) { } else if (point.X >= maxX || point.X <= minX) { } else { return; } g_Cells[point.Z % g_nSquaresInARow][point.Y % g_nSquaresInARow][point.X % g_nSquaresInARow] = true; } void Collide() { /* for (unsigned int iii = 0; iii < g_objects.size(); ++iii) { for (unsigned int jjj = 0; jjj < g_objects.size(); ++jjj) { if (iii == jjj) { continue; } } } */ } void Test() { MakeObjects(); InitCells(); unsigned int nMaxIters = 100; unsigned int nIters = 0; float fTimeElapsed = 0.0f; while (nIters < nMaxIters) { for (unsigned int iii = 0; iii < g_objects.size(); ++iii) { g_objects[iii].update(); BoundaryCheck(g_objects[iii].getPos()); Collide(); // TODO make objects collide // TODO process the g_Cells boolean values as instructions for VM } if (fTimeElapsed >= 5.0f) { g_objects.clear(); MakeObjects(); ++nIters; fTimeElapsed = 0.0f; } ResetCells(); fTimeElapsed = fTimeElapsed + 1.0f; } DeInitCells(); } int main() { Test(); return 0; } I want to clarify one thing. Assuming you had a game without physics, by adding physics you won't magically be better off. What i'm considering is what optimizations can be done for those games that already do have physics so we can recapture some of that. sure it would be cool to make games run on byproducts of simulation but IS NOT necessarily useful. But I just started this.
-
Hi I need your help ! And you can probably do it!
Prosper replied to Prosper's topic in Computer and Console
The source is not currently working for windows. -
Fact is I want my AI to synthesize dialog. The only step that is too much for me to complete is looking up words in an online dictionary then entering them and their usages into a database. I sure hope someone helps me. iI this database is done right, it won't take long for me to get the AI to say meaningful things even if not grammatically correct No I refuse to pay for some word database. None i've seen give me the usages in an easy to convert format. **** most word databases are just an incomplete list of words. Which brings me to how many do I need. I basically want to continue from what I have until there is no more words that need their usages defined . every usage might include a word that doesn't have an existence or usages. I have an existing database. Plenty of undefined words. and many words i have yet to define. as of now i have 428 words. although not all are defined. http://www.sendspace.com/file/i37qrn rename file to language.bin if it isn't already named that. You can view the database with a hex editor to get an idea of what exists. but you will need my software to add words. and ofcourse becareful. a few rules: no punctuation (use spaces instead) no abbreviation skip irrelevant details (things that could be removed from a defintion/usage without losing meaning of the rest) if you add a usage and the usage includes a word that does not exist the word that does not exist is replaced with something else (or even nothing). always double check a newly added usage to a word. Some commands: help (this will show you what you need to know) *the permutation commands aren't relevant at this time. Source code: http://codepad.org/3bzfp0dq A binary and source for linux (32 bit not 64 bit) http://www.sendspace.com/file/ke0mn8 *source should also be compatible for compile for windows might have to adjust project settings. protip: view the existing words and see how the usages are inputted. the source for which this project gets its words is dictionary.reference.com if anyone has the time make a server that can assign words that need to be defined. so we can avoid doing over the same things and work together. if you succeed in making the program i will give you a half priced EFPE. if anyone has the time make a server that can assign words that need to be defined. so we can avoid doing over the same things and work together. if you succeed in making the program i will give you a half priced EFPE. Bonus!!!! Extra Beginner's Guide for SUPER CLARITY set word and list word are commands you set a word if you want to : list its usages add new usages edit word's name (yes you can rename!) edit a word's usage (an index is required) if a word already exists it will tell you. if it does not exist it will not tell you. but you can observe a problem with the printout of a usage that uses a nonexisting word. you don't need to enter usages at the time you create a word. you should use 'set word' when you intend to edit it even if you just created it. new words aren't automatically selected.
-
http://i.imgur.com/kUv977Y.png http://i.imgur.com/bFeHFDw.png http://i.imgur.com/7TEt3uy.png pillars of eternity! http://i.imgur.com/TLSQ00e.png http://i.imgur.com/osZo5OU.png http://i.imgur.com/cPqdgGI.png
-
http://s28.postimg.org/geuki77zx/untitled.png http://s10.postimg.org/p7pey87p5/untitled.png http://i.imgur.com/wWVIdnR.png http://i.imgur.com/F3Cv1RD.png
-
http://i.imgur.com/w1sw6f1.png http://i.imgur.com/PAWjMiZ.png http://i.imgur.com/KByM1gB.png http://i.imgur.com/YdEEmYf.png http://i.imgur.com/OMEIVmj.gif http://i.imgur.com/dL6hisK.png http://i.imgur.com/nfAx0Ax.gif
-
http://i.imgur.com/OsPU3Zi.png